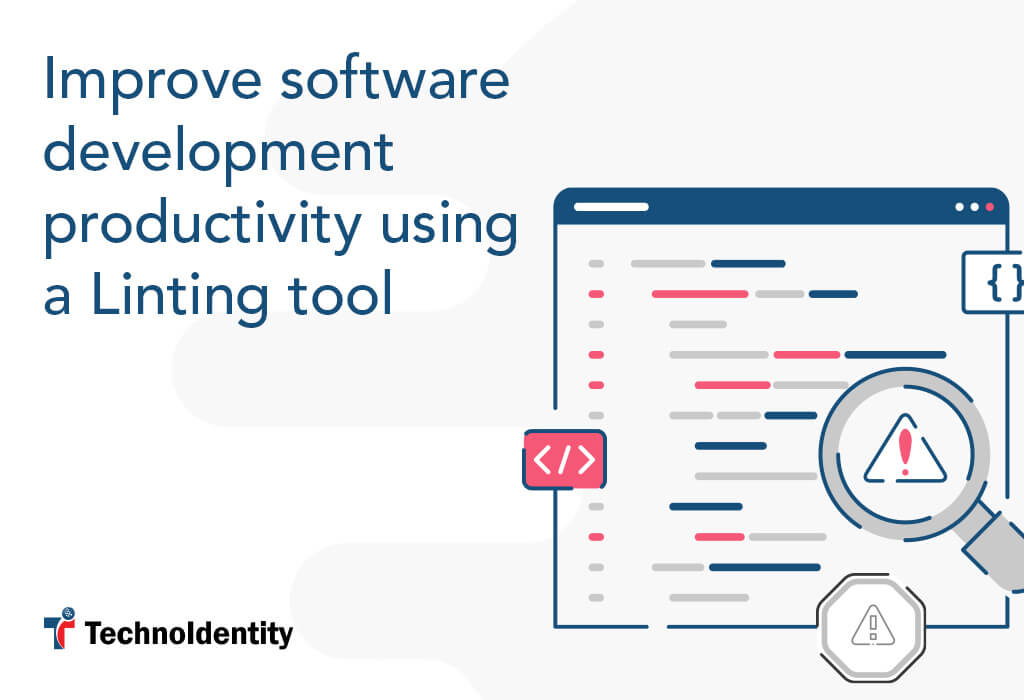
Improve software development productivity using a linting tool
What is a linting tool?
The first linting tool I ever used was for C & C++ programs, the main benefit for me being that it would point out parts of my program that weren’t portable or weren’t optimisabl by the compiler. Linting tools are available for all types of programming languages today and I would consider them a necessity because of the benefits it provides for little investment.
The feature set of linters these days overlaps somewhat with compilers and source code formatters but in general their core job is to help enforce uniform coding standards for your entire codebase. They perform static analysis of your source code and report questionable programming practices like:
- Common programming errors : using uninitialised variables, using assignment instead of equality operator in condition expressions
- Hard to maintain features : fall-through of `case` statements, using deprecated/outdated language features
- Bad practices : using magic numbers, using a mutable variable when const variable can be used
- Not adhering to a consistent coding style : naming functions inconsistently like getUserName & set_admin_password, using two vs. four spaces in different places
Some static analysis tools can do deeper analysis and report memory leaks, security vulnerabilities etc. If you want to know more about the kind of code smells a linting tool can alert you about, check out these guides:
Example style guides by Google
Example Javascript style guide by Airbnb
Benefits of using a linting tool
Linting tools are more effective than communicating best practices and coding guidelines orally to the team members & enforcing them via pull/merge requests. They help you keep the code clean, tidy, and at high quality, promoting clarity of mind of the programmers, which results in higher productivity. They help you write more maintainable, more secure, more robust and more efficient code.
They help experienced programmers transfer their knowledge of good practices to the entire team. Less experienced programmers can incrementally learn the best practices directly while they’re writing the code. As an example, when a programmer gets a linting error about unreachable code in their editor, they can go to the corresponding helpful page to learn why the tool is complaining about the code and what’s the recommended way of writing such code.
They are even more useful for dynamically-typed languages which don’t have a safety net in the form of a compiler. Javascript for example has a surprising amount of weird behaviour, though it has become a little better recently due to the standardisation efforts.
Because they help you notice and eliminate large number of common errors at editing/building phase itself, they help you cut down on the time spent on code reviews as well as writing a large number of unit tests.
Costs of using a linting tool
Cost 1: Creating the configuration file
Before you start using a linting tool you need to decide on a set of coding standards for your team and configure your linter tool to enforce them. This is generally done via a JSON/YAML type of text file.
Example ESLint config file:
You can create such a file from scratch or use/extend a popular, pre-existing config file commonly used by the community of your programming language, e.g.
Google’s config for linting Javascript code. You can always override specific rules that you don’t like in such a base config file.
You can always override specific rules that you don’t like in such a base config file.
Cost 2: Integration with CI/CD pipeline
If you’re using a linting tool it’s imperative that you make them a part of your CI pipeline. You want to make sure that the code written by any member of the team passes all the style/error checks of the linting tool before you integrate it with the rest of your code base. Doing this could be as simple as adding the command to lint your code after the build command/before the test command.
Example .gitlab-ci.yml code:
Cost 3: Integration with build tools
You need to add an extra step to your development workflow so you can analyse the code with a linting tool and fix the issues, just like you would fix the build errors. In some cases, you can fix the code that’s breaking the rules by simply running a command(e.g. lint –fix) or pressing a key-bind in your editor.
Running the lint tool as a Git hook makes sure that the programmers who’re pushing their code will find out any linter errors at commit/push time rather than as a result of a failed CI pipeline. This might seem unnecessary if developers make sure there are no linter errors before pushing the code but Git hooks can take the possibility of human error out of the equation. Use a tool like husky or pre-commit to make this step easier.
Example Husky configuration:
Cost 4: Integration with Editor/IDE like VS Code, Emacs, Vim, etc.
The preferred way to use a linter is by integrating it with your preferred editor. This way you’ll be alerted to the problematic piece of code as soon as you finish typing it; you can then immediately let the linter fix it for you in some cases, or you can fix it yourself manually.
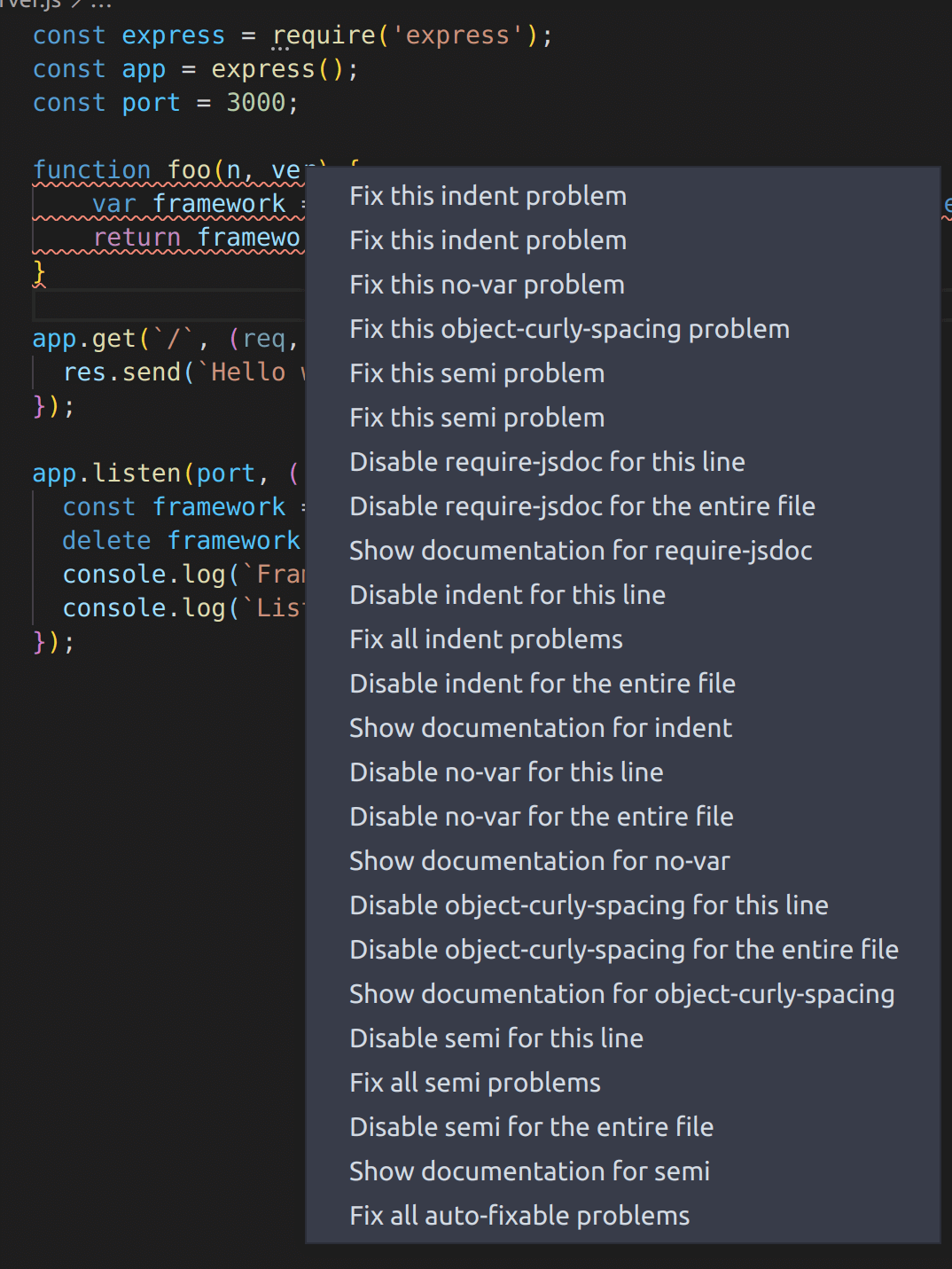
! Linter errors in Javascript code
Fixing all the linter errors can be overwhelming in the beginning for inexperienced programmers but this is actually one of the best ways to become a better programmer – write questionable code, get a linter warning, understand why the linter is complaining about it, and finally rewrite it following the recommended best practices. This isn’t really a cost of using a linter tool but rather a cost of learning good programming practices that’s made easier because of the linting tool.
To configure your editor with a linting tool, either search the name of the editor and linting tool together and follow the tutorials(e.g. emacs flake8) or go to the official web page of the linting tool and it should have configuration help for most popular editors. Examples:
Examples of linting tools
Linter | Programming language |
---|---|
checkstyle | Java |
pylint, flake8, pycodestyle | Python |
eslint | Javascript, Typescript |
csslint | Cascading Stylesheets |
rubocop | Ruby |
You can also go to Github’s collection of linters which lists most popular linting tools for various programming languages. You can also find a linter by searching the name of your programming language along with the
word linter, e.g. haskell linter.
Conclusion
Linting tools make your team be more productive by catching and fixing poorly written code early and reliably. It can be pretty easy to use them too if you
properly integrate them with your development tools. If you do the integration as part of creating a starter project of your preferred technology stack(or use
someone else’s starter project), you can re-use it for all of your future projects practically reducing the cost down to zero. I’ll show you how to do
exactly that for NodeJS based projects in a future post.